Hover over variable in the debugger to see the value either in the watch, local or editor windows.
This has been working great up until recently, I've started to get some infinite progress bars showing up like this:
The only way to fix it is to restart vs code.
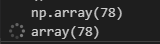
Traceback (most recent call last):
File "c:\Users\clovett\.vscode\extensions\ms-python.python-2019.5.18875\pythonFiles\lib\python\ptvsd\_vendored\pydevd\_pydevd_bundle\pydevd_frame.py", line 694, in trace_dispatch
self.do_wait_suspend(thread, frame, event, arg)
File "c:\Users\clovett\.vscode\extensions\ms-python.python-2019.5.18875\pythonFiles\lib\python\ptvsd\_vendored\pydevd\_pydevd_bundle\pydevd_frame.py", line 78, in do_wait_suspend
self._args[0].do_wait_suspend(*args, **kwargs)
File "c:\Users\clovett\.vscode\extensions\ms-python.python-2019.5.18875\pythonFiles\lib\python\ptvsd\_vendored\pydevd\pydevd.py", line 1460, in do_wait_suspend
keep_suspended = self._do_wait_suspend(thread, frame, event, arg, suspend_type, from_this_thread, frames_tracker)
File "c:\Users\clovett\.vscode\extensions\ms-python.python-2019.5.18875\pythonFiles\lib\python\ptvsd\_vendored\pydevd\pydevd.py", line 1482, in _do_wait_suspend
self.process_internal_commands()
File "c:\Users\clovett\.vscode\extensions\ms-python.python-2019.5.18875\pythonFiles\lib\python\ptvsd\_vendored\pydevd\pydevd.py", line 1199, in process_internal_commands
int_cmd.do_it(self)
File "c:\Users\clovett\.vscode\extensions\ms-python.python-2019.5.18875\pythonFiles\lib\python\ptvsd\_vendored\pydevd\_pydevd_bundle\pydevd_comm.py", line 496, in do_it
self.method(dbg, *self.args, **self.kwargs)
File "c:\Users\clovett\.vscode\extensions\ms-python.python-2019.5.18875\pythonFiles\lib\python\ptvsd\_vendored\pydevd\_pydevd_bundle\pydevd_comm.py", line 668, in internal_get_variable_json
for child_var in variable.get_children_variables(fmt=fmt):
File "c:\Users\clovett\.vscode\extensions\ms-python.python-2019.5.18875\pythonFiles\lib\python\ptvsd\_vendored\pydevd\_pydevd_bundle\pydevd_suspended_frames.py", line 114, in get_children_variables
dct = resolver.get_dictionary(self.value)
File "c:\Users\clovett\.vscode\extensions\ms-python.python-2019.5.18875\pythonFiles\lib\python\ptvsd\_vendored\pydevd\pydevd_plugins\extensions\types\pydevd_plugin_numpy_types.py", line 80, in get_dictionary
ret['[0:%s] ' % (len(obj))] = list(obj[0:MAX_ITEMS_TO_HANDLE])
IndexError: too many indices for array
PS D:\temp\yash> cd 'd:\temp\yash'; ${env:PYTHONIOENCODING}='UTF-8'; ${env:PYTHONUNBUFFERED}='1'; & 'C:\Anaconda3\envs\ell\python.exe' 'c:\Users\clovett\.vscode\extensions\ms-python.python-2019.5.18875\pythonFiles\ptvsd_launcher.py' '--default' '--client' '--host' 'localhost' '--port' '64847' 'd:\git\ELL\ELL\tools\importers\onnx\onnx_import.py' 'listener.onnx'
### Loading module C:\Anaconda3\envs\ell\lib\site-packages\pywin32_system32\pywintypes36.dll
### Loading module C:\Anaconda3\envs\ell\lib\site-packages\pywin32_system32\pythoncom36.dll
MainThread [2019-06-19 15:23:23,944] Pre-processing...
MainThread [2019-06-19 15:23:23,946] loading the ONNX model from: listener.onnx
MainThread [2019-06-19 15:23:23,994] Loaded ONNX model in 0.048 seconds.
MainThread [2019-06-19 15:23:23,995] ONNX IR_version 3
MainThread [2019-06-19 15:23:23,996] ONNX Graph producer: pytorch version 0.4
MainThread [2019-06-19 15:23:23,996] ONNX Graph total len: 25
MainThread [2019-06-19 15:23:24,012] Input 0 Inputs [] [] Outputs: ['0'] [((96, 1, 39), 'channel_row_column')] Attributes: {}
MainThread [2019-06-19 15:23:24,014] Constant 25 Inputs [] [] Outputs: ['25'] [((1,), 'channel')] Attributes: {'tensor': '...'}
MainThread [2019-06-19 15:23:24,015] Shape 26 Inputs ['0'] [((96, 1, 39), 'channel_row_column')] Outputs: ['26'] [((96, 1, 39), 'channel_row_column')] Attributes: {}
Traceback (most recent call last):
File "c:\Users\clovett\.vscode\extensions\ms-python.python-2019.5.18875\pythonFiles\lib\python\ptvsd\_vendored\pydevd\_pydevd_bundle\pydevd_frame.py", line 589, in trace_dispatch
self.do_wait_suspend(thread, frame, event, arg)
File "c:\Users\clovett\.vscode\extensions\ms-python.python-2019.5.18875\pythonFiles\lib\python\ptvsd\_vendored\pydevd\_pydevd_bundle\pydevd_frame.py", line 78, in do_wait_suspend
self._args[0].do_wait_suspend(*args, **kwargs)
File "c:\Users\clovett\.vscode\extensions\ms-python.python-2019.5.18875\pythonFiles\lib\python\ptvsd\_vendored\pydevd\pydevd.py", line 1460, in do_wait_suspend
keep_suspended = self._do_wait_suspend(thread, frame, event, arg, suspend_type, from_this_thread, frames_tracker)
File "c:\Users\clovett\.vscode\extensions\ms-python.python-2019.5.18875\pythonFiles\lib\python\ptvsd\_vendored\pydevd\pydevd.py", line 1482, in _do_wait_suspend
self.process_internal_commands()
File "c:\Users\clovett\.vscode\extensions\ms-python.python-2019.5.18875\pythonFiles\lib\python\ptvsd\_vendored\pydevd\pydevd.py", line 1199, in process_internal_commands
int_cmd.do_it(self)
File "c:\Users\clovett\.vscode\extensions\ms-python.python-2019.5.18875\pythonFiles\lib\python\ptvsd\_vendored\pydevd\_pydevd_bundle\pydevd_comm.py", line 496, in do_it
self.method(dbg, *self.args, **self.kwargs)
File "c:\Users\clovett\.vscode\extensions\ms-python.python-2019.5.18875\pythonFiles\lib\python\ptvsd\_vendored\pydevd\_pydevd_bundle\pydevd_comm.py", line 668, in internal_get_variable_json
for child_var in variable.get_children_variables(fmt=fmt):
File "c:\Users\clovett\.vscode\extensions\ms-python.python-2019.5.18875\pythonFiles\lib\python\ptvsd\_vendored\pydevd\_pydevd_bundle\pydevd_suspended_frames.py", line 114, in get_children_variables
dct = resolver.get_dictionary(self.value)
File "c:\Users\clovett\.vscode\extensions\ms-python.python-2019.5.18875\pythonFiles\lib\python\ptvsd\_vendored\pydevd\pydevd_plugins\extensions\types\pydevd_plugin_numpy_types.py", line 80, in get_dictionary
ret['[0:%s] ' % (len(obj))] = list(obj[0:MAX_ITEMS_TO_HANDLE])
IndexError: too many indices for array
Traceback (most recent call last):
File "c:\Users\clovett\.vscode\extensions\ms-python.python-2019.5.18875\pythonFiles\ptvsd_launcher.py", line 43, in <module>
main(ptvsdArgs)
File "c:\Users\clovett\.vscode\extensions\ms-python.python-2019.5.18875\pythonFiles\lib\python\ptvsd\__main__.py", line 434, in main
run()
File "c:\Users\clovett\.vscode\extensions\ms-python.python-2019.5.18875\pythonFiles\lib\python\ptvsd\__main__.py", line 312, in run_file
runpy.run_path(target, run_name='__main__')
File "C:\Anaconda3\envs\ell\lib\runpy.py", line 263, in run_path
pkg_name=pkg_name, script_name=fname)
File "C:\Anaconda3\envs\ell\lib\runpy.py", line 96, in _run_module_code
mod_name, mod_spec, pkg_name, script_name)
File "C:\Anaconda3\envs\ell\lib\runpy.py", line 85, in _run_code
exec(code, run_globals)
File "d:\git\ELL\ELL\tools\importers\onnx\onnx_import.py", line 95, in <module>
main()
File "d:\git\ELL\ELL\tools\importers\onnx\onnx_import.py", line 91, in main
convert(args.input, args.output_directory, args.zip_ell_model, args.step_interval, args.lag_threshold)
File "d:\git\ELL\ELL\tools\importers\onnx\onnx_import.py", line 42, in convert
lag_threshold_msec=lag_threshold)
File "d:\git\ELL\ELL\tools\importers\onnx\onnx_to_ell.py", line 32, in convert_onnx_to_ell
importer_model = converter.load_model(path)
File "d:\git\ELL\ELL\tools\importers\onnx\lib\onnx_converters.py", line 1684, in load_model
return self.set_graph(graph)
File "d:\git\ELL\ELL\tools\importers\onnx\lib\onnx_converters.py", line 1706, in set_graph
node = self.get_converter(onnx_node).convert(onnx_node)
File "d:\git\ELL\ELL\tools\importers\onnx\lib\onnx_converters.py", line 119, in convert
node.output_shapes = self.get_output_shapes()
File "d:\git\ELL\ELL\tools\importers\onnx\lib\onnx_converters.py", line 750, in get_output_shapes
t = self.gather_tensor(self.node) # actually do it...
File "d:\git\ELL\ELL\tools\importers\onnx\lib\onnx_converters.py", line 778, in gather_tensor
self.add_tensor(node.id, t)
File "d:\git\ELL\ELL\tools\importers\onnx\lib\onnx_converters.py", line 778, in gather_tensor
self.add_tensor(node.id, t)
File "c:\Users\clovett\.vscode\extensions\ms-python.python-2019.5.18875\pythonFiles\lib\python\ptvsd\_vendored\pydevd\_pydevd_bundle\pydevd_frame.py", line 589, in trace_dispatch
self.do_wait_suspend(thread, frame, event, arg)
File "c:\Users\clovett\.vscode\extensions\ms-python.python-2019.5.18875\pythonFiles\lib\python\ptvsd\_vendored\pydevd\_pydevd_bundle\pydevd_frame.py", line 78, in do_wait_suspend
self._args[0].do_wait_suspend(*args, **kwargs)
File "c:\Users\clovett\.vscode\extensions\ms-python.python-2019.5.18875\pythonFiles\lib\python\ptvsd\_vendored\pydevd\pydevd.py", line 1460, in do_wait_suspend
keep_suspended = self._do_wait_suspend(thread, frame, event, arg, suspend_type, from_this_thread, frames_tracker)
File "c:\Users\clovett\.vscode\extensions\ms-python.python-2019.5.18875\pythonFiles\lib\python\ptvsd\_vendored\pydevd\pydevd.py", line 1482, in _do_wait_suspend
self.process_internal_commands()
File "c:\Users\clovett\.vscode\extensions\ms-python.python-2019.5.18875\pythonFiles\lib\python\ptvsd\_vendored\pydevd\pydevd.py", line 1199, in process_internal_commands
int_cmd.do_it(self)
File "c:\Users\clovett\.vscode\extensions\ms-python.python-2019.5.18875\pythonFiles\lib\python\ptvsd\_vendored\pydevd\_pydevd_bundle\pydevd_comm.py", line 496, in do_it
self.method(dbg, *self.args, **self.kwargs)
File "c:\Users\clovett\.vscode\extensions\ms-python.python-2019.5.18875\pythonFiles\lib\python\ptvsd\_vendored\pydevd\_pydevd_bundle\pydevd_comm.py", line 668, in internal_get_variable_json
for child_var in variable.get_children_variables(fmt=fmt):
File "c:\Users\clovett\.vscode\extensions\ms-python.python-2019.5.18875\pythonFiles\lib\python\ptvsd\_vendored\pydevd\_pydevd_bundle\pydevd_suspended_frames.py", line 114, in get_children_variables
dct = resolver.get_dictionary(self.value)
File "c:\Users\clovett\.vscode\extensions\ms-python.python-2019.5.18875\pythonFiles\lib\python\ptvsd\_vendored\pydevd\pydevd_plugins\extensions\types\pydevd_plugin_numpy_types.py", line 80, in get_dictionary
ret['[0:%s] ' % (len(obj))] = list(obj[0:MAX_ITEMS_TO_HANDLE])
IndexError: too many indices for array
[Extension Host] Info Python Extension: 2019-06-19 15:08:28: Cached data exists getEnvironmentVariables, d:\git\ELL\ELL\tools\importers\onnx\lib\onnx_converters.py
3workbench.main.js:238 [Extension Host] Info Python Extension: 2019-06-19 15:09:18: Cached data exists getEnvironmentVariables, d:\git\ELL\ELL\tools\importers\onnx\lib\onnx_converters.py
workbench.main.js:238 [Extension Host] Warn Python Extension: 2019-06-19 15:09:18: Linter 'pylint' is not installed. Please install it or select another linter". [Error: Module 'pylint' not installed.
at p.execModule (C:\Users\clovett\.vscode\extensions\ms-python.python-2019.5.18875\out\client\extension.js:83:293115)]
t.log @ workbench.main.js:238
3workbench.main.js:238 [Extension Host] Info Python Extension: 2019-06-19 15:09:22: Cached data exists getEnvironmentVariables, d:\git\ELL\ELL\tools\importers\onnx\lib\onnx_converters.py
workbench.main.js:238 [Extension Host] Warn Python Extension: 2019-06-19 15:09:23: Linter 'pylint' is not installed. Please install it or select another linter". [Error: Module 'pylint' not installed.
at p.execModule (C:\Users\clovett\.vscode\extensions\ms-python.python-2019.5.18875\out\client\extension.js:83:293115)]
t.log @ workbench.main.js:238
2workbench.main.js:238 [Extension Host] Info Python Extension: 2019-06-19 15:09:23: Cached data exists getEnvironmentVariables, d:\git\ELL\ELL\tools\importers\onnx\onnx_import.py
workbench.main.js:238 [Extension Host] Info Python Extension: 2019-06-19 15:09:28: Cached data exists getEnvironmentVariables, d:\git\ELL\ELL\tools\importers\onnx\lib\onnx_converters.py
workbench.main.js:238 [Extension Host] Info Python Extension: 2019-06-19 15:18:59: Cached data exists getEnvironmentVariables, tasks
workbench.main.js:238 [Extension Host] Info Python Extension: 2019-06-19 15:19:03: Cached data exists getEnvironmentVariables, extension-output-#2
workbench.main.js:238 [Extension Host] Info Python Extension: 2019-06-19 15:19:21: Cached data exists getEnvironmentVariables, extension-output-#1
workbench.main.js:238 [Extension Host] Info Python Extension: 2019-06-19 15:19:28: Cached data exists getEnvironmentVariables, rendererLog
workbench.main.js:238 [Extension Host] Info Python Extension: 2019-06-19 15:19:44: Cached data exists getEnvironmentVariables, d:\git\ELL\ELL\tools\importers\onnx\lib\onnx_converters.py
workbench.main.js:238 [Extension Host] Info Python Extension: 2019-06-19 15:19:55: Cached data exists getEnvironmentVariables, sharedLog
workbench.main.js:238 [Extension Host] Info Python Extension: 2019-06-19 15:20:02: Cached data exists getEnvironmentVariables, extension-output-#4
workbench.main.js:238 [Extension Host] Info Python Extension: 2019-06-19 15:20:04: Cached data exists getEnvironmentVariables, extension-output-#2
workbench.main.js:238 [Extension Host] Info Python Extension: 2019-06-19 15:20:36: Cached data exists getEnvironmentVariables, d:\git\ELL\ELL\tools\importers\onnx\lib\onnx_converters.py