Access your 1Password items in your Go applications through your self-hosted 1Password Connect server.
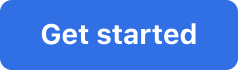
The 1Password Connect Go SDK provides access to the 1Password Connect API, to facilitate communication with the Connect server hosted on your infrastructure and 1Password. The library is intended to be used by your applications, pipelines, and other automations to simplify accessing items stored in your 1Password vaults.
-
Download and install the 1Password Connect Go SDK:
go get github.com/1Password/connect-sdk-go
-
Export the
OP_CONNECT_HOST
andOP_CONNECT_TOKEN
environment variables:export OP_CONNECT_HOST=<your-connect-host> && \ export OP_CONNECT_TOKEN=<your-connect-token>
-
Use it in your code
-
Read a secret:
import "github.com/1Password/connect-sdk-go/connect" func main () { client := connect.NewClientFromEnvironment() item, err := client.GetItem("<item-uuid>", "<vault-uuid>") if err != nil { log.Fatal(err) } }
-
Write a secret:
import ( "github.com/1Password/connect-sdk-go/connect" "github.com/1Password/connect-sdk-go/onepassword" ) func main () { client := connect.NewClientFromEnvironment() item := &onepassword.Item{ Title: "Secret String", Category: onepassword.Login, Fields: []*onepassword.ItemField{{ Value: "mysecret", Type: "STRING", }}, } postedItem, err := client.CreateItem(item, "<vault-uuid>") if err != nil { log.Fatal(err) } }
-
For more examples, check out USAGE.md.
- File an issue for bugs and feature requests.
- Join the Developer Slack workspace.
- Subscribe to the Developer Newsletter.
1Password requests you practice responsible disclosure if you discover a vulnerability.
Please file requests via BugCrowd.
For information about security practices, please visit the 1Password Bug Bounty Program.