lager is a C++ library to assist value-oriented design by implementing the unidirectional data-flow architecture. It is heavily inspired by Elm and Redux, and enables composable designs by promoting the use of simple value types and testable application logic via pure functions. And you get time-travel for free!
- Documentation (Contents)
- Code (GitHub)
- CppRussia-Piter 2019 Talk: Squaring the circle (YouTube, Slides)
- CppCon 2018 Talk: The most valuable values (YouTube, Slides)
- C++ on Sea 2019 Talk: Postmodern immutable data-structures (YouTube, Slides)
![]()
This project is part of a long-term vision helping interactive and concurrent C++ programs become easier to write. Help this project's long term sustainability by becoming a patron or buying a sponsorship package: [email protected]
For a guided introductory tour with code samples, please read the architecture overview section. Other examples:
- Counter, a minimalistic example with multiple UIs (link).
- Autopong, a basic game using SDL2 (link).
- Ewig, a terminal text editor with undo, asynchronous loading, and more (link).
Most interactive software of the last few decades has been written using an object-oriented interpretation of the Model View Controller design. This architecture provides nice separation of concerns, allowing the core application logic to be separate from the UI, and a good sense of modularity. However, its reliance on stateful object graphs makes the software hard to test or parallelize. It's reliance on fine-grained callbacks makes composition hard, resulting in subtle problems that are hard to debug.
Value-based unidirectional data-flow tackles a few of these problems:
- Thanks to immutability and value-types, it is very easy to add concurrency as threads can operate on their local copies of the data without mutexes or other flaky synchronization mechanisms. Instead, worker threads communicate their results back by dispatching actions to the main thread.
- The application logic is made of pure functions that can be easily tested and are fully reproducible. They interact with the world via special side-effects procedures loosely coupled to the services they need via dependency injection.
- This also means that data and call-graphs are always trees or DAGs (instead of cyclical graphs), with explicit composition that is to trace and debug. You can also always snapshot the state, making undo and time-travel easy peasy!
This library is written in C++17 and a compliant compiler and standard library necessary. It is continuously tested with GCC 7, but it might work with other compilers and versions.
It also depends on Zug and Boost Hana. Some optional extensions and modules may have other dependencies documented in their respective sections.
This is a header only you can just copy the lager
subfolder
somewhere in your include path.
Some components, like the time-travelling debugger, also require the installation of extra files.
You can use CMake to install the library in your system once you have manually cloned the repository:
mkdir -p build && cd build cmake .. && sudo make install
In order to develop the library, you will need to compile and run the examples, tests and benchmarks. These require some additional tools. The easiest way to install them is by using the Nix package manager. At the root of the repository just type:
nix-shell
This will download all required dependencies and create an isolated environment in which you can use these dependencies, without polluting your system.
Then you can proceed to generate a development project using CMake:
mkdir build && cd build cmake ..
From then on, one may build and run all tests by doing:
make check
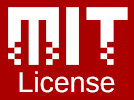
This software is licensed under the MIT license.
The full text of the license is can be accessed via this link and is also included in the
LICENSE
file of this software package.