when i was run this scrip it:
`import time
from cryptography.hazmat.primitives.asymmetric.ed25519 import Ed25519PrivateKey
from libra import stdlib, utils, libra_types, testnet, AuthKey
from libra.testnet import CHAIN_ID
CURRENCY = "Coin1"
"""
submit_peer_to_peer_transaction_example demonstrates currencies transfer between 2 accounts on the Libra blockchain
"""
def main():
# connect to testnet
client = testnet.create_client()
# generate private key for sender account
sender_private_key = Ed25519PrivateKey.generate()
# generate auth key for sender account
sender_auth_key = AuthKey.from_public_key(sender_private_key.public_key())
print(f"Generated sender address: {utils.account_address_hex(sender_auth_key.account_address())}")
# create sender account
faucet = testnet.Faucet(client)
**testnet.Faucet.mint(faucet, sender_auth_key.hex(), 100000000, "Coin1")**
# get sender account
sender_account = client.get_account(sender_auth_key.account_address())
# generate private key for receiver account
receiver_private_key = Ed25519PrivateKey.generate()
# generate auth key for receiver account
receiver_auth_key = AuthKey.from_public_key(receiver_private_key.public_key())
print(f"Generated receiver address: {utils.account_address_hex(receiver_auth_key.account_address())}")
# create receiver account
faucet = testnet.Faucet(client)
testnet.Faucet.mint(faucet, receiver_auth_key.hex(), 10000000, CURRENCY)
# create script
script = stdlib.encode_peer_to_peer_with_metadata_script(
currency=utils.currency_code(CURRENCY),
payee=receiver_auth_key.account_address(),
amount=10000000,
metadata=b'', # no requirement for metadata and metadata signature
metadata_signature=b'',
)
# create transaction
raw_transaction = libra_types.RawTransaction(
sender=sender_auth_key.account_address(),
sequence_number=sender_account.sequence_number,
payload=libra_types.TransactionPayload__Script(script),
max_gas_amount=1_000_000,
gas_unit_price=0,
gas_currency_code=CURRENCY,
expiration_timestamp_secs=int(time.time()) + 30,
chain_id=CHAIN_ID,
)
# sign transaction
signature = sender_private_key.sign(utils.raw_transaction_signing_msg(raw_transaction))
public_key_bytes = utils.public_key_bytes(sender_private_key.public_key())
signed_txn = utils.create_signed_transaction(raw_transaction, public_key_bytes, signature)
# submit transaction
client.submit(signed_txn)
# wait for transaction
client.wait_for_transaction(signed_txn)
if name == "main":
main()
`
it error
Traceback (most recent call last):
File "D:/work/libra-python-test/main.py", line 71, in
main()
File "D:/work/libra-python-test/main.py", line 25, in main
testnet.Faucet.mint(faucet, sender_auth_key.hex(), 100000000, "Coin1")
File "C:\Python38\lib\site-packages\libra\testnet.py", line 66, in mint
self._retry.execute(lambda: self._mint_without_retry(authkey, amount, currency_code))
File "C:\Python38\lib\site-packages\libra\jsonrpc\client.py", line 86, in execute
raise e
File "C:\Python38\lib\site-packages\libra\jsonrpc\client.py", line 80, in execute
return fn()
File "C:\Python38\lib\site-packages\libra\testnet.py", line 66, in
self._retry.execute(lambda: self._mint_without_retry(authkey, amount, currency_code))
File "C:\Python38\lib\site-packages\libra\testnet.py", line 85, in _mint_without_retry
self._client.wait_for_transaction(txn)
File "C:\Python38\lib\site-packages\libra\jsonrpc\client.py", line 425, in wait_for_transaction
return self.wait_for_transaction2(
File "C:\Python38\lib\site-packages\libra\jsonrpc\client.py", line 460, in wait_for_transaction2
txn = self.get_account_transaction(address, seq, True)
File "C:\Python38\lib\site-packages\libra\jsonrpc\client.py", line 320, in get_account_transaction
return self.execute("get_account_transaction", params, _parse_obj(lambda: rpc.Transaction()))
File "C:\Python38\lib\site-packages\libra\jsonrpc\client.py", line 491, in execute
return self._retry.execute(
File "C:\Python38\lib\site-packages\libra\jsonrpc\client.py", line 80, in execute
return fn()
File "C:\Python38\lib\site-packages\libra\jsonrpc\client.py", line 492, in
lambda: self.execute_without_retry(method, params, result_parser, ignore_stale_response)
File "C:\Python38\lib\site-packages\libra\jsonrpc\client.py", line 523, in execute_without_retry
json = self._rs.send_request(self, request, ignore_stale_response or False)
File "C:\Python38\lib\site-packages\libra\jsonrpc\client.py", line 98, in send_request
return client._send_http_request(client._url, request, ignore_stale_response)
File "C:\Python38\lib\site-packages\libra\jsonrpc\client.py", line 554, in _send_http_request
self.update_last_known_state(
File "C:\Python38\lib\site-packages\libra\jsonrpc\client.py", line 262, in update_last_known_state
if curr.version > version:
TypeError: '>' not supported between instances of 'int' and 'NoneType'
Process finished with exit code 1
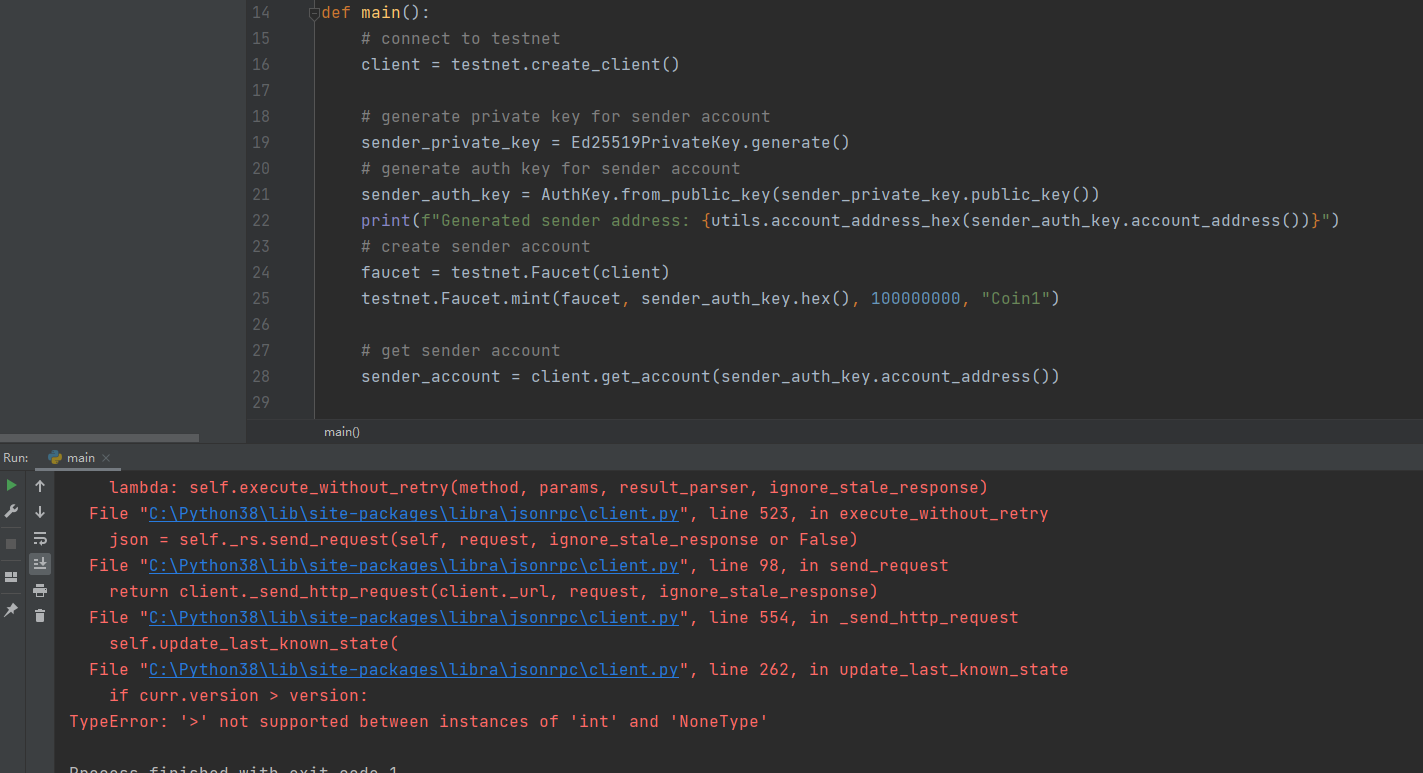
it ok last monday!